Creating Social Posts with the ChatGPT API

[ad_1]
If you don’t live under a metaphorical rock technology you have heard of or tried ChatGPT. You know, good OpenAI for good conversation. Since its launch in November 2022, ChatGPT has only been available through a GUI interface – and when we say “available” we mean the OpenAPI development language model “gpt-3.5-turbo” that powers ChatGPT. Other, less dramatic, linguistic examples exist. However, recently OpenAI released the promised ChatGPT API which allows you to call “gpt-3.5-turbo” via the API. This, dear friends, opens up a world of new applications including ChatGPT created social media posts.
Why Use the ChatGPT API for Social Media?
There are several reasons why you might want to integrate the ChatGPT API into your environment or application:
User Create Content on Your Platform
Your users create content, such as photos or videos, on your site and share it on social media, but the point of contention is writing social content. For example, your creator’s marketplace produces an amazing photo of a cat sitting on a shoe – you’re promoting the shoes, not the cat – and you have a button to share the photo of the cat in the shoe on Instagram.
But what text do you want to add to that post, and what hashtags? Are you making the user type it or are you using template text. There are no good solutions.
ChatGPT is Hot Now
Everyone is talking about ChatGPT and integrating AI into your platform is a must-do, and it gives some public companies a stock boost when they announce AI integration. It may sound like following the crowd, but it is really useful for your user and clients. If you can remove friction, increase customer satisfaction, and possibly increase revenue, do it.
Generating Social Media Post text using the ChatGPT API
Let’s get into the fun stuff and generate social media posts with text from the ChatGPT API and send them to Instagram Facebook using Ayrshare’s social media APIs. Note that we will be using an AI generated image using DALL·E.
Get the Chat API key
The first step is to sign up for an OpenAI account and generate an API key. Just click on your profile icon in the top right corner and select “View API keys”.
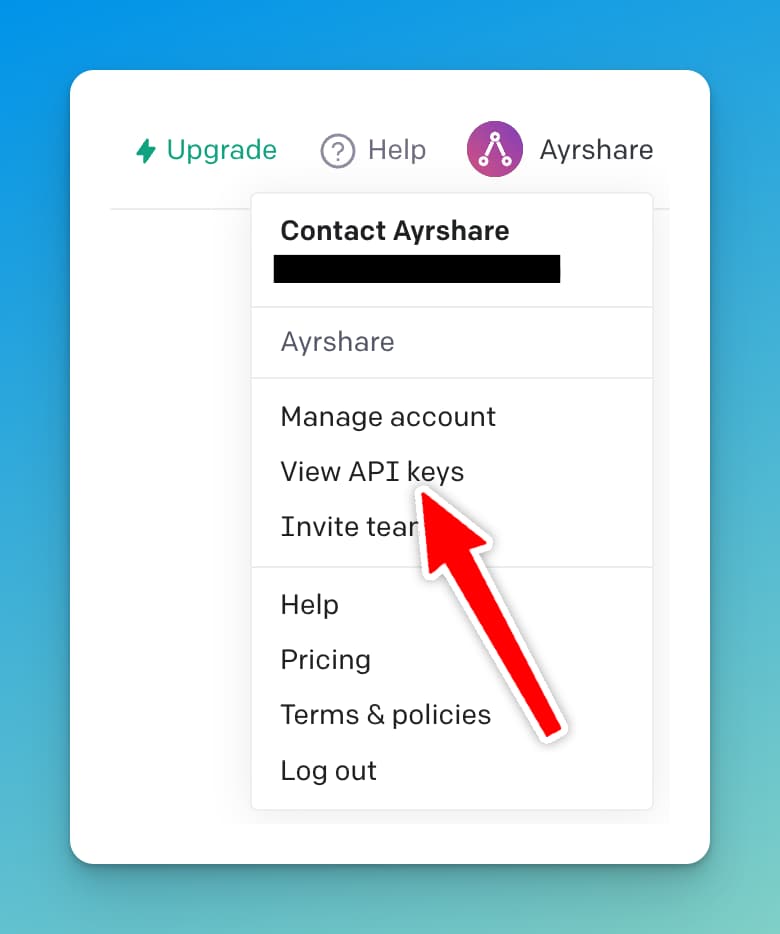
Signing up for Open AI is free and you get a large amount of credits to try things out.
Call the ChatGPT API Endpoint
With our API key in hand, let’s make our first API endpoint call. Every request on ChatGPT should have a request, such as “I wrote a blog post on the likes of the TV show Yellowstone”. In our example, we will use the text “Write a social media post about a cat sitting on a shoe”. We will also use the GPT-3 language model. However, we recommend using the latest language model, GPT-4, as it provides better results.
Here is an example of cURL:
curl
-H "Authorization: Bearer $OPENAI_API_KEY"
-H "Content-Type: application/json"
-d '{
"model": "gpt-3.5-turbo",
"messages": [{"role": "user", "content": "What is the OpenAI mission?"}]
}'
We’ll use JavaScript examples going forward, but since these are REST calls you can write them in your favorite programming language, like Python or PHP.
const OPENAI_API_KEY = "Your API OpenAI Key";
const run = async () => {
const options = {
method: "POST",
headers: {
Authorization: `Bearer ${OPENAI_API_KEY}`,
"Content-Type": "application/json",
},
body: JSON.stringify({
model: "gpt-3.5-turbo",
messages: [
{
role: "user",
content: "Write a social media post on a cat sitting in a shoe",
},
],
}),
};
const chatGPTResults = await fetch(
"",
options
).then((res) => res.json());
console.log("ChatGPT says:", JSON.stringify(chatGPTResults, null, 2));
};
run();
What does this code do? Detailed instructions can be found in the OpenAI documentation, but here’s a quick summary.
- SEND calls to URL
for ChatGPT API requests.
Authorization
: Include the OpenAI API key in the header.model
: Include in the post body the name of the model, which is ChatGPTgpt-3.5-turbo
.role
: Orsystem
,user
orassistant
. We want to useuser
as we are asking for something.content
: A question or request we make to ChatGPT.
Result of the call:
{
"id": "chatcmpl-6ruxjLkNNtIKeZ0dPdCGG2ijXIYHd",
"object": "chat.completion",
"created": 1678308367,
"model": "gpt-3.5-turbo-0301",
"usage": {
"prompt_tokens": 19,
"completion_tokens": 56,
"total_tokens": 75
},
"choices": [
{
"message": {
"role": "assistant",
"content": "nnThis adorable little kitten has found a new favorite spot to lounge around in! Can you guess what it is? Yep, you got it- a shoe! How cute is this? 😍😻 #catinshoe #kittenlove #cutenessoverload"
},
"finish_reason": null,
"index": 0
}
]
}
ChatGPT Assistant responds to content
field:
nnThe adorable little cat has found a new favorite place to hang out! Can you guess what it is? Yes, you got it- a shoe! How good is this? 😍😻 #catinshoe #kittenlove #cutenessoverload
Not only is this a great summary, but it has emojis and hashtags. If you call the endpoint again, you will get a different result.
Posting on Social Networks
Now that we have our post script, let’s publish the post. We will be using Ayrshare’s public API and you can sign up for a free account to test it out. After registration, get your Ayrshare API key, the last key you need to get, we promise, you can connect other public accounts in the Public Accounts tab, such as Facebook, Instagram, and Twitter.
We will update our code so that we can now take the generated text and send it to social media using the cat in the shoe image.
const OPENAI_API_KEY = "Your API OpenAI Key";
const AYRSHARE_API_KEY = "Your API Ayrshare Key";
const run = async () => {
const options = {
method: "POST",
headers: {
Authorization: `Bearer ${OPENAI_API_KEY}`,
"Content-Type": "application/json",
},
body: JSON.stringify({
model: "gpt-3.5-turbo",
messages: [
{
role: "user",
content: "Write a social media post on a cat sitting in a shoe",
},
],
}),
};
const chatGPTResults = await fetch(
"",
options
).then((res) => res.json());
console.log("ChatGPT says:", JSON.stringify(chatGPTResults, null, 2));
const optionsPost = {
method: "POST",
headers: {
Authorization: `Bearer ${AYRSHARE_API_KEY}`,
"Content-Type": "application/json",
},
body: JSON.stringify({
post: chatGPTResults.choices[0].message.content,
platforms: ["facebook", "instagram"],
mediaUrls: ["
}),
};
const postResults = await fetch(
"
optionsPost
).then((res) => res.json());
console.log("Ayrshare says:", JSON.stringify(postResults, null, 2));
};
run();
Response from ChatGPT and Ayrshare:
ChatGPT says: {
"id": "chatcmpl-6rvrDplRIwdiUURwXzdU3iS0KiYUo",
"object": "chat.completion",
"created": 1678311807,
"model": "gpt-3.5-turbo-0301",
"usage": {
"prompt_tokens": 19,
"completion_tokens": 80,
"total_tokens": 99
},
"choices": [
{
"message": {
"role": "assistant",
"content": "nn"Who needs a cozy bed when you have a shoe! 😺 This little furry feline has found the perfect spot to curl up and take a cat nap. Can you blame them? That shoe looks so snug and comforting. 🥰 What crazy places have you found your cats napping in?" #catsittinginshoes #crazycatnaps #furryfriends"
},
"finish_reason": null,
"index": 0
}
]
}
Ayrshare says: {
"status": "success",
"errors": [],
"postIds": [
{
"status": "success",
"id": "738681876342836_1098688181078083",
"postUrl": "
"platform": "facebook"
},
{
"status": "success",
"id": "17987746003883214",
"postUrl": "
"usedQuota": 1,
"platform": "instagram"
}
],
"id": "KRRormDnpBTgfKYadpmn",
"refId": "d93ca2784c9bf7bf83ce0bf081d16c91598aec28",
"post": "nn"Who needs a cozy bed when you have a shoe! 😺 This little furry feline has found the perfect spot to curl up and take a cat nap. Can you blame them? That shoe looks so snug and comforting. 🥰 What crazy places have you found your cats napping in?" #catsittinginshoes #crazycatnaps #furryfriends"
}
And here are the original posts on Instagram and Facebook:
The New Social World
We’ve seen how easy it is to create content and publish to the community using APIs. This is just the beginning of what can be done when you combine AI-generated content with automated social posting.
If you want to learn more about how ChatGPT works or the social media APIs check out these articles:
Generate Social Posts and Rewrite Posts
If you’re looking for an endpoint that handles both creating social posts or rewriting posts – social networks don’t like duplicate posts – then try our new ChatGPT enabled / enable API endpoint.
The API handles the creation, formatting, adding hashtags and emojis, and even making sure that the post has the required length, i.e. Twitter and 280 characters.