API Design Best Practices

[ad_1]
Tips for Creating Well-Designed and Maintainable APIs
At Ayrshare, we continue to see Application Programming Interfaces (APIs) being adopted as the backbone of seamless communication between different software applications. And like a good (dad) joke, an API should be easy to understand and leave a lasting impression. You don’t want engineers scratching their heads and saying, “I don’t get it!” – translation “I’m out of here.”
A well-designed API ensures a great developer experience, leading to happy users and happy clients.
In this article, we’ll discuss tips and guidelines for designing APIs with some examples from Ayrshare’s REST API endpoints.
Understand your developer audience
Before writing a single line of code, it is important that know who will use your API. Are they experienced developers or newcomers? Understanding your target audience influences decisions about complexity, style of text, and level of reach. Think about your own use case – what do you want when you read a company’s API documentation, e.g. Stripe.
At Ayrshare, we’ve designed our API to be both simple and flexible, creating something we love to use ourselves. Whether you’re a beginner developer who wants to quickly integrate social media deployments or an enterprise that needs advanced features like analytics and user management, our API offers a wide range of standards and requirements.
Establish Compliance with Standards
Consistency is key to API design. Do you want to match different levels, for example some response APIs use camelCase and others snake case (snake_case)? And in the same data different field names are used like age and user age? Yuck.
By having a consistent standard, you establish a meaningful contract with your users and reduce the learning curve, frustration, and hopefully prevent errors.
When establishing API consistency and standards focus on:
- HTTP methods: Stick to RESTful principles by using HTTP methods correctly:
GET
to return servicesPOST
creating resourcesPUT
orPATCH
for reviewing servicesDELETE
by removing resources
- The answer Field Names: If one last answer is
userId
do not useid
in the other endpoint response if it has the same value. - Data formats: Stick to standard data formats such as Zulu time to ensure consistency and universality.
- Structure of the endpoint URL: Many API philosophies exist about how to structure an endpoint URL, for example
GET
https://api.ayrshare.com/api/analytics
. In this example the verbGET
and typeanalytics
. It doesn’t matter if this is your style in comparisonGET
https://api.ayrshare.com/api/getAnalytics
l The important point is consistency in all points. - HTTP Response Codes: The HTTP response code is the first indication to the API user that the call was successful or if a problem occurred. For example, a response in the 200 range means that everything is fine. A response of 400 or higher means there is a problem.
Examples:
Creating Posts: To create new social media posts, Ayrshare uses i POST
the way with /post
conclusion:
POST https://api.ayrshare.com/api/post
Body of the Request:
{
"post": "Hello World!",
"platforms": ["facebook"]
}
Answer Body:
{
"status": "success",
"errors": [],
"postIds": [
{
"status": "success",
"id": "1835773643315433893",
"postUrl": "https://www.facebook.com/33892",
"platform": "facebook"
}
],
"id": "G5y3PRI7bzLq1GmV5Xaa",
"refId": "9abf1426d6ce9122ef11c72bd62e59807c5cc092",
"post": "A beautiful day",
"validate": true
}
Consider returning frequently a status
like "error"
or "success"
and make sure it matches the HTTP response code.
Receiving Posts: To retrieve information for a specific post, use the GET
the way with /post/{id}
conclusion:
GET https://app.ayrshare.com/api/post/1234567890
This consistent use of HTTP methods and endpoint structures makes the API simple and easy to use.
Design with scalability in mind
As your API grows, it must handle the increased load without performance degradation.
- Statelessness: Design your API to be stateless. Each request must contain all the information needed to be processed, allowing for better ranking. Each Ayrshare API request includes all necessary information, such as API keys and parameters, ensuring that the server does not need to maintain session information between requests.
- Effective Resource Management: Optimize your points to handle multiple tasks efficiently. If all goes well, you’ll be getting a lot of API calls and you need to be able to support them all. Consider a serverless infrastructure – such as Supabase or Firebase – to automatically handle the additional load, but be warned that autoscaling can be expensive, so be sure to set budget limits.
- Load Balancing and Rate Limiting: Use strategies to spread the load and prevent abuse. In order to maintain efficiency and prevent abuse, Ayrshare uses a fair use measure, detailed in our documentation, to ensure fair use among all customers. Bring it back
429
The HTTP response code if there was a rate limit error. - Working with Batches: Ayrshare allows you to send posts to multiple social networks with a single request, reducing the number of API calls. This is good for your user and your system.
{
"post": "Exciting news! Our app just got better.",
"platforms": ["twitter", "facebook", "linkedin", "instagram"]
}
Use a Clear Cancellation Policy
As your API evolves, certain features or endpoints may not work. Using a clear deprecation policy ensures that changes to your API don’t break existing integrations and gives developers enough time to adapt.
- Advance Notice: Communicate upcoming changes early. This can be done through email notifications, dashboard alerts, or dedicated announcements.
- Literature Review: Clearly mark the deprecated elements in your documents, including the date of deprecation and the planned deletion date.
- Times of Grace: Provide reasonable time frames when supporting both old and new features, allowing developers to switch smoothly.
- Other Solutions: Whenever possible, suggest alternative solutions or methods that developers can implement in place of retired features.
If we plan to withdraw a storage space or feature from Ayrshare, we will announce it by:
- Email Communications: Subscribers receive information in the monthly newsletter about changes, including deadlines.
- Literature Review: Deprecated features are clearly stated in our documentation, with instructions for alternatives. This is on the upcoming API changes page.
- Time of Grace: We ensure that deprecated features remain active for a significant period of time, usually several months, to give developers enough time to update their apps. Sometimes social networks only give 60 days, but in those cases we can try to find another way or notify our users right away.
- Support During Transition: Our support team is available to help developers migrate to new features or repositories, ensuring minimal disruption to their services.
By focusing on a strong withdrawal policy, Ayrshare maintains a stable and reliable API environment, as we introduce new features and improvements.
Prioritize Security Measures
Protecting data and ensuring secure communication is non-negotiable. DO NOT NEGOTIATE!
Authentication and Authorization: Use strong authentication methods such as API keys or OAuth 2.0. Ayrshare uses Bearer API keys for authentication. Enter your API key in Authorization
header:
Authorization: Bearer YOUR_API_KEY
HTTPS Everywhere: Force HTTPS to encrypt data during transit. Ayrshare endpoints require HTTPS, ensuring that all data transmitted is encrypted.
Safety Check: Perform regular PEN (Penetration Testing) on your APIs. If you don’t try to break them, someone else will.
Provide Clear and Complete Documentation
Good documentation is as important as the API itself.
API reference: Detailed information about each endpoint, parameters, application examples and responses is essential. A lot of information is good as long as it is organized. Here is an example of our post endpoint:
Tutorials and Instructions: Provide step-by-step guides for common tasks to help developers get started quickly. A written lesson is great, but a video lesson will make you shine! For example, here is our video guide on Make a connection:
Code samples: Examples in many programming languages. AI today is very good at translating from one language to another, but people still like to see examples in their preferred language and make it nice and helpful. For example:
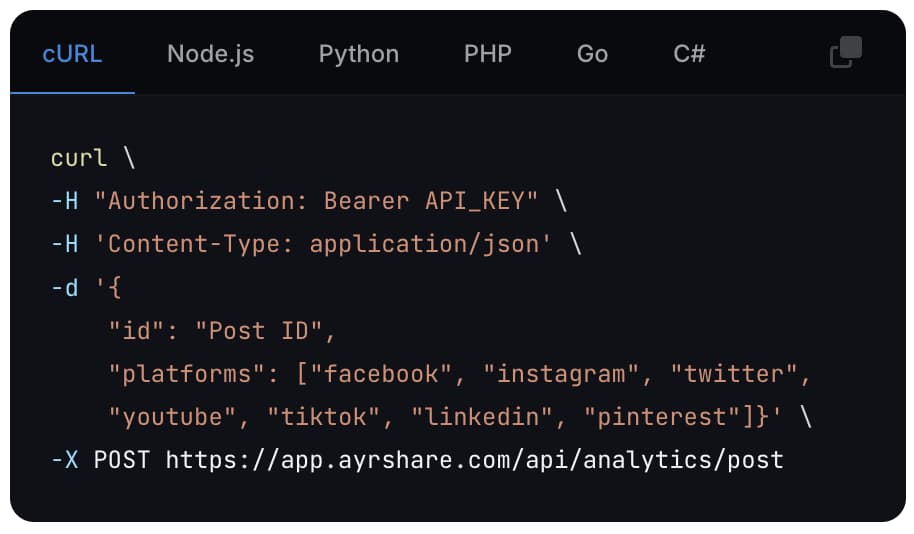
Use Strong Error Handling
Clear and consistent error handling tools for debugging and improving the developer experience.
- Common HTTP Status Codes: Use appropriate status codes to display the result of an API call. Please see above for discussion.
- Descriptive Error Messages: Provide logical error messages and codes to help identify and resolve problems.
- Structure of the Error Object: Maintain a consistent format for error responses.
For example, here is the error for deleting a post when the submitted post id could not be found:
HTTP/1.1 400 Bad Request
Content-Type: application/json
{
"action": "delete",
"status": "error",
"code": 114,
"message": "Delete id not found.",
"id": "IdpUOyihLEpIx0d0N9mI"
}
Configure Simple Testing and Monitoring
Ensure that your API is reliable and works well under various conditions.
- Automated Testing: Use unit and integration tests to catch problems early. We’re big fans of Hurl for automating our API testing and recommend it to our users.
- Monitoring Tools: Use monitoring utilities to track API performance and timing. We use this to run our internal monitoring and to send status updates to customers.
- Logging: Keep detailed logs for testing and troubleshooting purposes. This is important for customer support. A good logging program will make your life easier, trust us.
- Internal Tools: As your business grows, you will find the need for tools to support your API users. Our favorite intern platform is Retool.
- External Inspection: Ultimately your users need to be able to test your API. We recommend Postman and also provide a Postman JSON file with all our predefined endpoints – it makes calls really easy.
At Ayrshare, we understand the importance of strong API design. Our Social Media API is built with these best practices in mind, ensuring seamless integration and a better developer experience.